Unreal Engine 4
Toon Tanks
Final Product: Sound, Particles, HUD, Level Creation
The HUD was created using Blueprint Implementable Events, where a function with the UFUNCTION property BlueImplementableEvent is called in code, and the body of the code is done in Blueprints. The HUD consists of the player's health, and the number of enemies left.
Another part of the HUD and the game that was added were winning and losing conditions, in which the screen would display if you had won by defeating all the enemy towers, or lost because of the players health hitting 0. To also add to more immersion, Camera Shake was added when firing to give a more intense feeling to the game.
The environment was created with assets from the free Unreal Marketplace environment pack created by Julio Juarez found in the link below. Below that link, you can find a download link to play the game yourself!
Julio Juarez's Polar Sci-Fi Facility Environment Pack: Unreal Marketplace
Game Link Download: Google Drive
Update 3: Firing and Health
The first was to get the projectile to detect when a hit would occur. This would be done using a multicast delegate variable that an actor inherits, called OnComponentHit. This was done by assigning a callback function with the proper parameters and binding it to the delegate.
The second step would be to actually have a health component so that damage would come through. This was also done with a multicast delegate. But first, I had created an actor component in C++ called HealthComponent. The delegate that was used was a variable called OnTakeAnyDamage, and a callback function was assigned to it to apply the damage. The place where the delegate would be told to broadcast, however, was done in the projectile class, whenever OnHit occurred.
Below is the current culmination of the project so far in video form. If you look at the console, you can see where the health is actually being subtracted whenever a projectile lands on either a tower or the tank.
Update 2: Character Movement


For the turret to rotate itself towards the player tank, I simply enabled ticking on the enemy tower, and it would check each tick if the player tank was in its range (an editable variable). If it was, it would call a function that would rotate the turret.
Though, I noticed that there was a commonality there as well, so I moved the RotateTurret function into BasePawn so both tank and tower would have access to it. The way the function would work, is that it would get a LookAtTarget (simply a vector in the world), get the turret mesh's location, subtract the two to get a vector with the correct direction and magnitude, and apply that to the turrets rotation.
For moving the tank's turret, it would get the mouse cursors position based off a HitResult returned by the player controller.
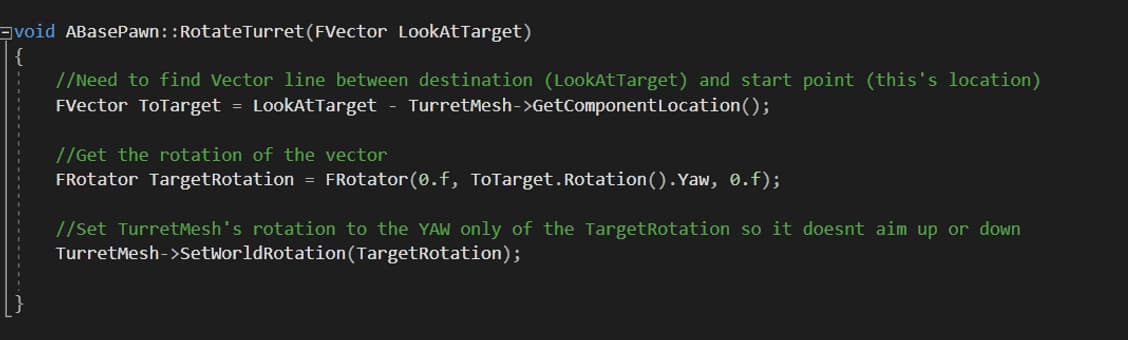
Start: Character Creation in C++
The commonalities between the two are that they will need a capsule for collision detection, a base, a turret, and a position where the projectile being shot would spawn in. I made gave these all the UPROPERTY's of being only visible, so that the pointers themselves could not be changed in the Unreal Editor.
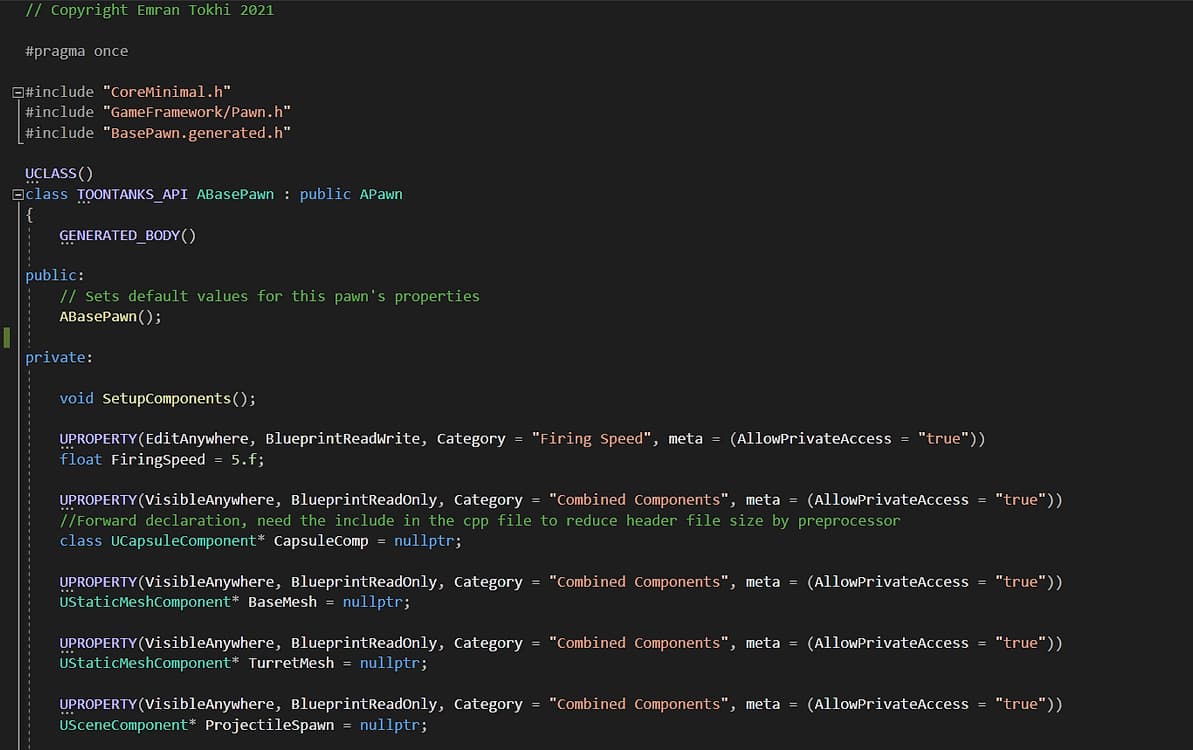
After doing so, my focus moved to creating the Tank class, a child of the BasePawn. For this class, I also needed a camera component that would be used for the player's view. With that came a spring arm that the camera is always attached to. There was also an enemy tower class that was created. (The rest of the code can be found on my GitHub.)
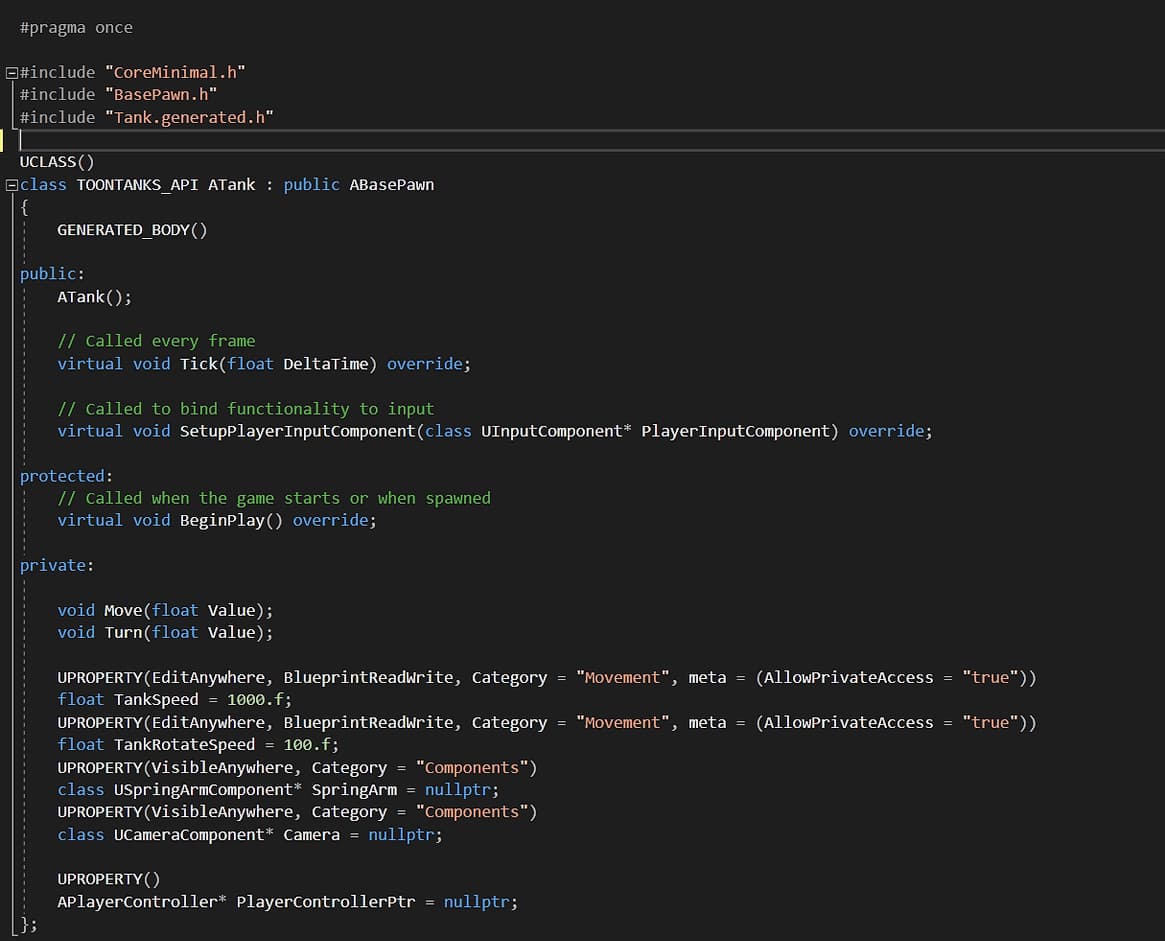
After creating the classes, I was ready to create blueprints for the tank and the turret. I made blueprints for both and based both on their respective parent classes. Once that was done, I was able to assign each component that was required in the blueprint as shown.

